In the world of coding, selecting a programming paradigm is a decision with far-reaching effects. The chosen approach shapes everything from code readability to scalability and maintainability, ultimately influencing a project’s success and adaptability.
Programming paradigms—such as object-oriented, functional, and imperative—each have unique strengths, making the choice highly contextual. While some paradigms are well-suited to data manipulation and others to modelling complex entities, understanding when and why to apply each style is essential for creating clean, effective, and purpose-driven code.
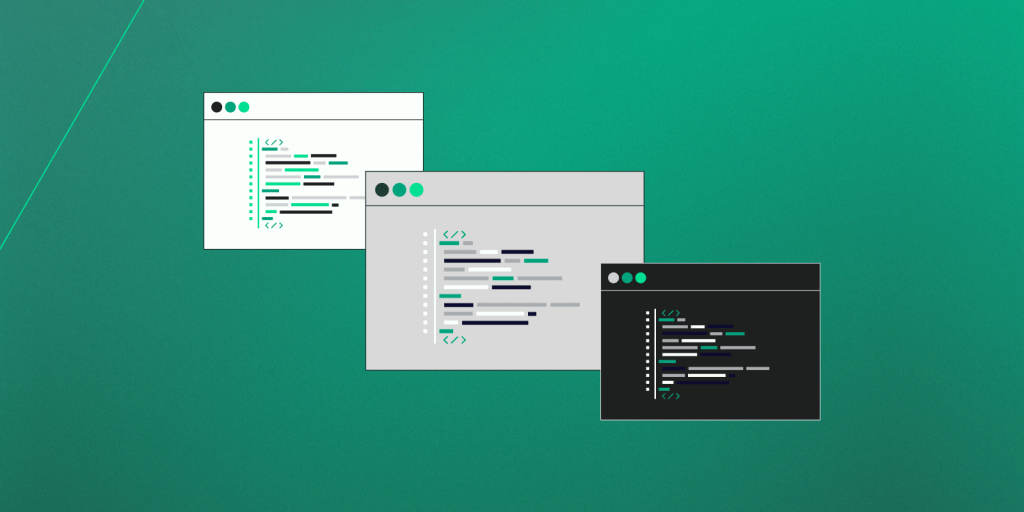
A Balanced View of Programming Paradigms
Programming paradigms generally fall into four main categories: object-oriented, functional, imperative, and declarative. Each has its strengths and ideal use cases:
– Object-oriented programming (OOP): Centres around objects and data models, often mapping naturally to real-world entities.
– Functional programming: Emphasizes immutability, pure functions, and treats computation as the evaluation of mathematical functions.
– Imperative programming: Focuses on sequences of commands that change a program’s state, which aligns well with the procedural nature of many web applications.
– Declarative programming: It’s ideal for tasks that benefit from high-level instructions, abstracting away the procedural steps.
When to Use Functional Programming
Functional programming offers a powerful approach to coding, mainly when reliability, testability, and clear data handling are top priorities. Its emphasis on immutability, pure functions, and predictable outcomes makes it an excellent choice for scenarios where modular and reusable code is essential. Functional programming allows developers to simplify complex data transformations, handle user interactions with ease, and reduce unintended side effects, which can enhance applications’ overall stability and maintainability.
Functional programming is particularly effective for:
– Data Transformation and Processing: Functional programming is well-suited for manipulating and transforming data, especially in applications that rely on complex data flows. Pure functions allow for predictable outputs based on inputs, making data operations easy to test and maintain.
– Stateless Logic: Functional programming’s emphasis on immutability is highly effective for operations that don’t rely on application state, such as filtering posts, sorting data, or formatting content. Since functions don’t change external state, they reduce unexpected interactions and simplify debugging.
– Event Handling and User Interactions: Functional programming can help manage event handlers cleanly and predictably in user-facing applications. Functional approaches make it easier to compose small, reusable functions that respond to user interactions without altering the state directly.
– Concurrency and Parallelism: Functional programming’s immutability makes it naturally suited for concurrent applications where tasks run in parallel. By avoiding shared states, functional code reduces the risk of race conditions, making it a practical choice for high-performance systems with concurrent processes.
– Reusable and Composable Code: Functional programming encourages writing smaller, reusable functions that can be easily composed together to perform more complex operations. This approach enhances code modularity and allows developers to create versatile functions that can be repurposed across different parts of an application.
When to Use Imperative Programming
Imperative programming excels in tasks that require a precise, ordered sequence of instructions to achieve a specific result. This paradigm is particularly effective when each step builds on the previous one, making it a strong choice for scripting, configuration, and automation tasks.
In web development and CMS environments, imperative programming is well-suited for:
– Workflow Automation: Tasks like deployment scripts, data migrations, and automated backups benefit from an imperative, step-by-step approach. Each command directly impacts the system’s state, allowing precise control over the operation flow.
– State Management: Imperative programming directly updates the state for applications with simple or predictable state changes, such as setting user preferences or toggling UI elements. This immediate feedback makes it easier to track and debug.
– Procedural Logic in CMS Platforms: Many CMS systems, like WordPress, use imperative programming to define core application logic. Handling form submissions, executing database queries, or setting up routes is often best done imperatively, giving developers direct control over the sequence and error handling.
– Command-driven Applications: Applications that rely on command input, such as CLI tools, benefit from imperative programming by processing each command as received. This allows the code to respond directly to user actions and guide users through ordered processes.
When to Use Declarative Programming
Declarative programming is a powerful approach for defining what the result should look like without detailing how to achieve it. This style is particularly effective in scenarios where predictable, consistent output is essential and when working with complex UIs or data structures. By focusing on the desired end state rather than the step-by-step logic, declarative programming simplifies code, improves readability, and often makes maintenance easier.
In general, declarative programming is effective for:
– User Interface Development: Declarative programming excels in UI frameworks like React, where components represent the desired state of the interface. Developers specify what each part of the UI should display, and the framework manages the underlying changes, making it easier to build responsive, dynamic interfaces.
– Styling and Layouts with CSS and HTML: CSS is inherently declarative, allowing developers to describe how elements should look. With layout frameworks like Flexbox and Grid, developers can define layouts declaratively without writing explicit positioning code.
– Data Querying and Management: In database querying languages like SQL, declarative programming lets developers specify what data they need rather than how to retrieve it. Similarly, declarative tools for data binding or state management allow developers to set rules for data flow and updating, making complex data handling more manageable.
– Configuration and Infrastructure as Code: Tools like Terraform and CloudFormation use declarative configurations to define infrastructure. By stating the desired infrastructure state, these tools automatically handle provisioning and adjustments, simplifying cloud deployments.
Using Object-Oriented Programming Judiciously
At Trew Knowledge, we approach programming flexibly and open-mindedly, recognizing that different projects and code requirements call for different strategies. While our preference often leans towards functional and imperative approaches, we don’t rigidly follow one paradigm over another. Instead, we tailor our choices to the unique demands of each project, drawing from object-oriented programming (OOP) when it makes the most sense, especially when dealing with real-world entities or scenarios that naturally lend themselves to an object-based model.
For instance, in a content management environment, a “Post” object might carry properties like title, author, and published date, making it well-suited for OOP. However, we avoid more intricate OOP patterns, such as factories or singletons, unless the problem truly warrants it.
Ultimately, our approach is to keep code as simple and efficient as possible. By refraining from applying OOP by default, we aim to prevent unnecessary complexity. In cases where object-oriented methods do add value, we implement them thoughtfully and sparingly.
Pragmatism Over Rigid Adherence
One of the key pitfalls in development is adopting coding patterns without fully understanding their purpose—a phenomenon often called cargo-cult programming. This occurs when developers implement certain styles or patterns simply because they’re popular, without questioning whether they fit the problem best.
Consider a scenario where a developer might use a singleton pattern in WordPress to manage a global configuration class. While this may work, it often introduces complexity without adding much value. Instead, we advocate for using such patterns only when they serve a clear purpose.
Cargo-cult programming can be avoided by solidly understanding each paradigm’s strengths and limitations. Rather than defaulting to a particular style, it’s essential to consider factors such as performance, ease of testing, and code readability.
The Best Approach for WordPress Development
WordPress development benefits most from a pragmatic blend of programming paradigms tailored to its inherently procedural, PHP-based architecture. Since WordPress core is primarily built with imperative and procedural code, adopting a hybrid approach that combines functional, imperative, and selectively applied object-oriented programming (OOP) can yield the most efficient and maintainable results.
Here’s a look at why this mix works well and how to apply it in practice:
– Imperative Approach for Core Interactions: Given WordPress’s procedural foundation, imperative programming is well-suited for tasks that manipulate posts, users, and settings.
– Functional Programming for Data Manipulation: Functional programming is highly effective for filtering posts, processing form data, or formatting content.
– Selective Object-Oriented Programming (OOP): WordPress is not inherently object-oriented, so it’s best to use it only where it adds clear value—typically with entities representing real-world objects or repeatable behaviours. For instance, creating classes for custom post types, users, or plugins can encapsulate functionality in a manageable way.
– Declarative Approach for Modern Block Development: When working with the block editor (Gutenberg), a declarative approach, similar to JavaScript frameworks, is useful. The block editor allows developers to focus on what content should look like rather than how to structure it. Using JavaScript’s declarative style to create reusable, dynamic components enhances the overall user experience and aligns with modern web standards. Declarative programming can also streamline how WordPress themes handle styling and layout. Using CSS and WordPress’s theme.json file, developers can define appearance rules declaratively, ensuring a consistent look and feel while reducing the need for repetitive code.
Tips for Making Informed Decisions on Programming Approaches
1. Prioritize Readability and Maintainability
Clear, readable code is crucial, especially when working in collaborative environments or on long-term projects. While using advanced patterns or complex constructs may be tempting, simplicity often leads to better maintainability. Before implementing any design pattern, ask: Will this code be easy for someone else (or me, in a few months) to understand and maintain?
2. Consider the Context of Your Development Environment
Each development environment has its own quirks, dependencies, and best practices. Aim to work with the environment rather than against it, choosing patterns and paradigms that fit naturally within its ecosystem.
3. Optimize for Performance, but Only When Necessary
Certain paradigms and patterns may impact performance, especially in resource-constrained environments. Functional programming, for example, emphasizes immutability, which can increase memory use if not handled carefully. Object-oriented approaches can sometimes add overhead due to their structure. While performance is essential, it’s also important to avoid premature optimization. Focus on writing clean, functional code first, and then fine-tune for performance if profiling shows it’s necessary.
4. Balance Modularity with Simplicity
Modularity is a great asset—it helps isolate functionality and promotes reusability. However, it’s important not to break code into too many small pieces, especially if those pieces don’t have a clear, distinct purpose. Striking a balance between modular design and simplicity can reduce developers’ cognitive load and keep the codebase streamlined.
5. Test for Flexibility and Extensibility
Regardless of which paradigm you choose, keeping your code flexible and easy to extend is vital. As projects evolve, new features or requirements will inevitably arise. Designing your code to accommodate change will save time and effort in the long run. Using clean, modular patterns with clear interfaces can help create a more adaptable codebase.
6. Document Decisions and Rationale
Documenting what you’ve implemented and why you made those choices is essential. In cases where a less common paradigm or pattern is applied, documenting your rationale can guide future developers and help maintain continuity. This is particularly helpful in collaborative environments, as it records the team’s thought process and aligns everyone’s understanding.
7. Embrace Testing as Part of Development
Testing isn’t just about finding bugs; it’s a way to validate that each piece of your code fulfills its intended purpose. You can confirm that your chosen paradigms and patterns work harmoniously together by incorporating automated testing, such as unit tests, integration tests, or even behavioural tests. This is especially crucial when integrating multiple paradigms within a single project.
Commitment to Tailored Solutions
At Trew Knowledge, purpose-driven coding is at the heart of our programming philosophy. Our client-centric approach ensures that every solution we deliver is built on a foundation of technical excellence and perfectly tailored to meet and exceed the unique needs of those we serve.
By aligning our technical strategies with business objectives, we create not only code but value, fostering long-term relationships and ongoing success for our clients. Ready to see the difference the proper programming can make? Connect with our experts today.